Graphics Supplement (Part 1)
Savitch 1.4, 2.5, 3.4, 4.3
Graphics Supplement 1-4: Outline
JavaFX
- JavaFX is a set of packages that allow Java programmers to create graphics and media applications.
- 2D, 3D games
- Visual Effects
- Touch-enabled applications
- Successor to AWT and Swing for making graphical applications
- In JavaFX you add scenes to the stage. A _canvas is an object that you can draw shapes on.
A Sample JavaFX Application
- View sample program Listing 1.2 class
HappyFace
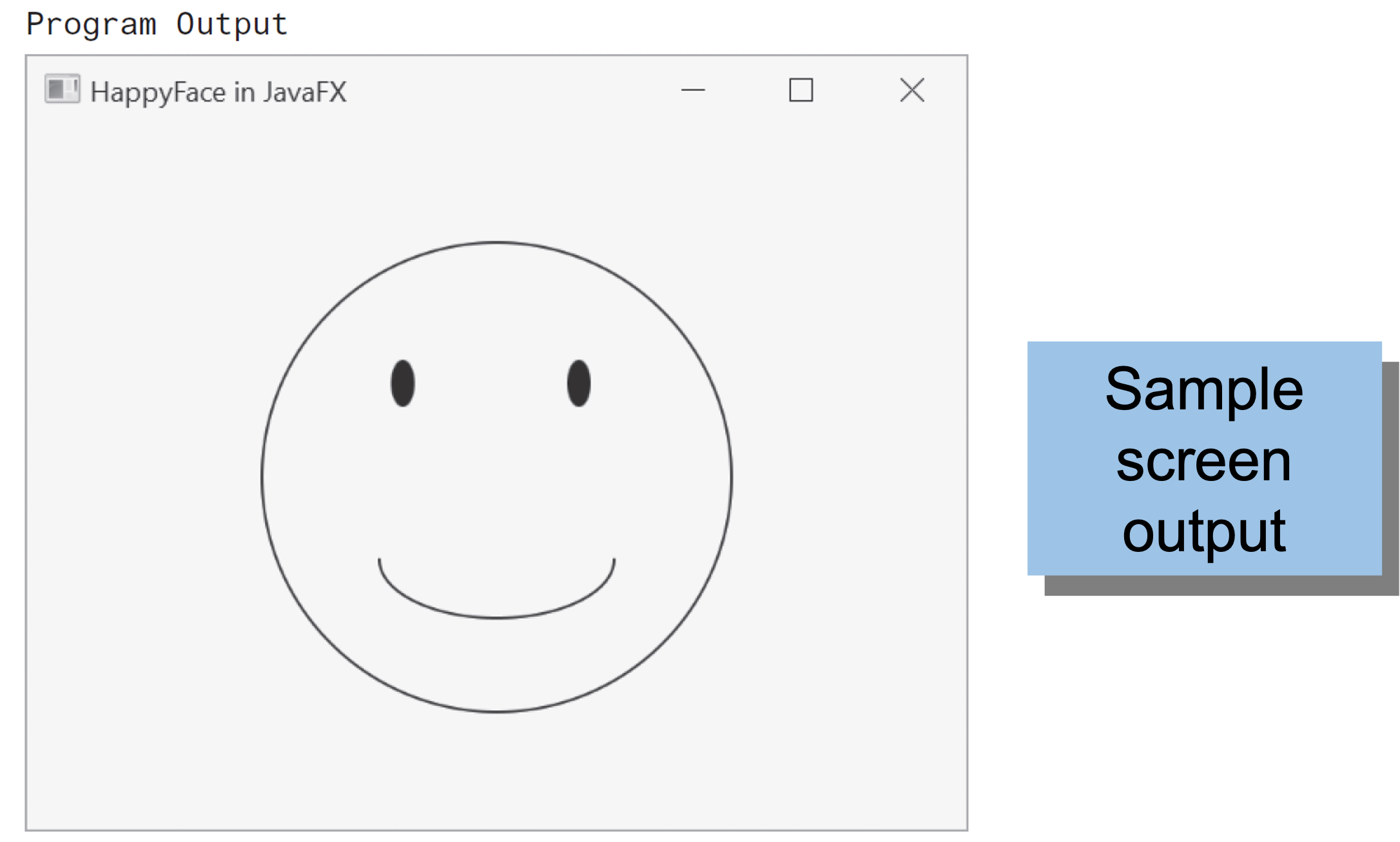
- The
start
method is the starting method for a JavaFX application, not the main method
- The first four lines of the
start
method set up a canvas on a scene for you to draw simple graphics
Screen Coordinate System
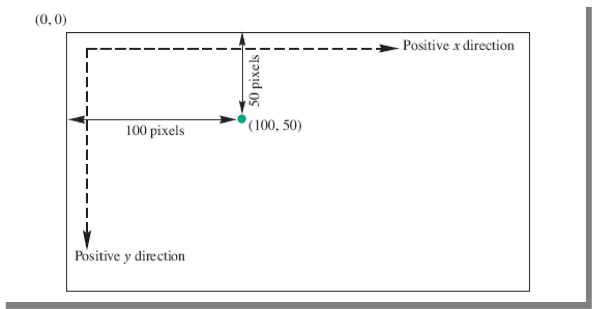
- The x-coordinate is the number of pixels from the from the left.
- The y-coordinate is the number of pixels from the top (not from the bottom).
Drawing Ovals and Circles
- The
strokeOval
method draws only the outline of the oval.
gc.strokeOval(100, 50, 90, 50);
- The
fillOval
method draws a filled-in oval.
gc.fillOval(100, 50, 90, 50);
- The
strokeOval
and fillOval
methods take four arguments.
- The first two arguments indicate the upper-left corner of an invisible rectangle around the oval.
- The last two arguments indicate the width and height of the oval.
- A circle is just an oval whose height is the same as its width.
- Figure 1.7 The Oval Drawn by
gc.strokeOval(100, 50, 90, 50)
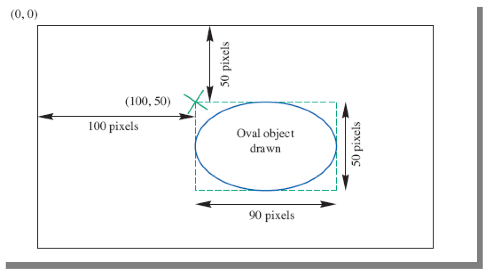
- Sizes and positions in a JavaFX program are given in pixels.
- Think of the display surface for the applet as being a two-dimensional grid of individual pixels.
Drawing Arcs
- The
strokeArc
method draws an arc.
strokeArc(100, 50, 200, 200, 180, 180, ArcType.OPEN);
- The
strokeArc
method takes seven arguments.
- The first four arguments are the same as the four arguments needed by the
strokeOval
method.
- The next two arguments indicate where the arc starts, and the number of degrees through which is sweeps.
- O degrees is horizontal and to the right.
- The last argument indicates if the arc is open or closed.
Specifying an Arc
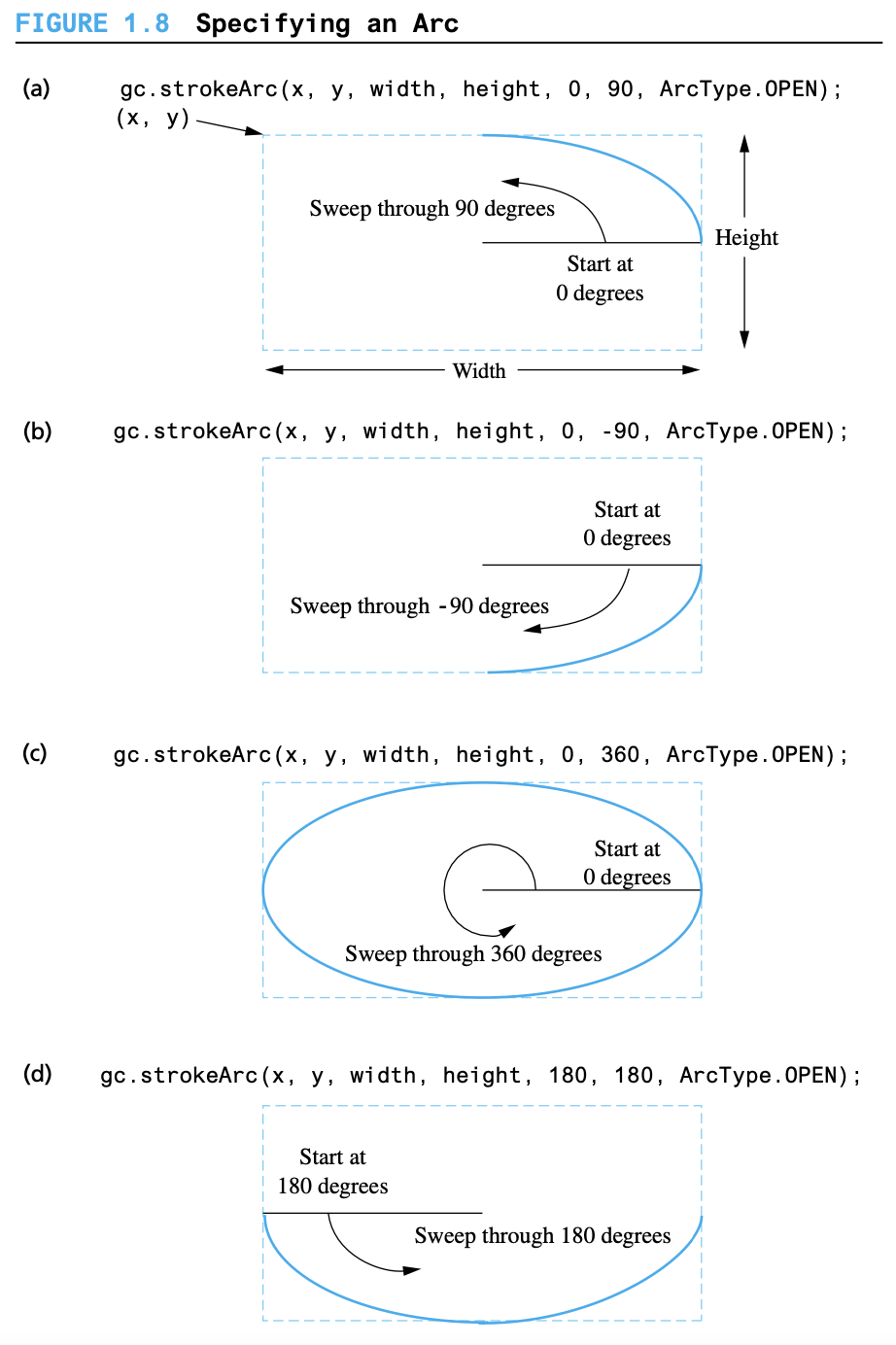
Graphics Supplement: Outline Section 2.5
Style Rules Applied to a JavaFX Application
- View sample program class
HappyFace
listing 2.9
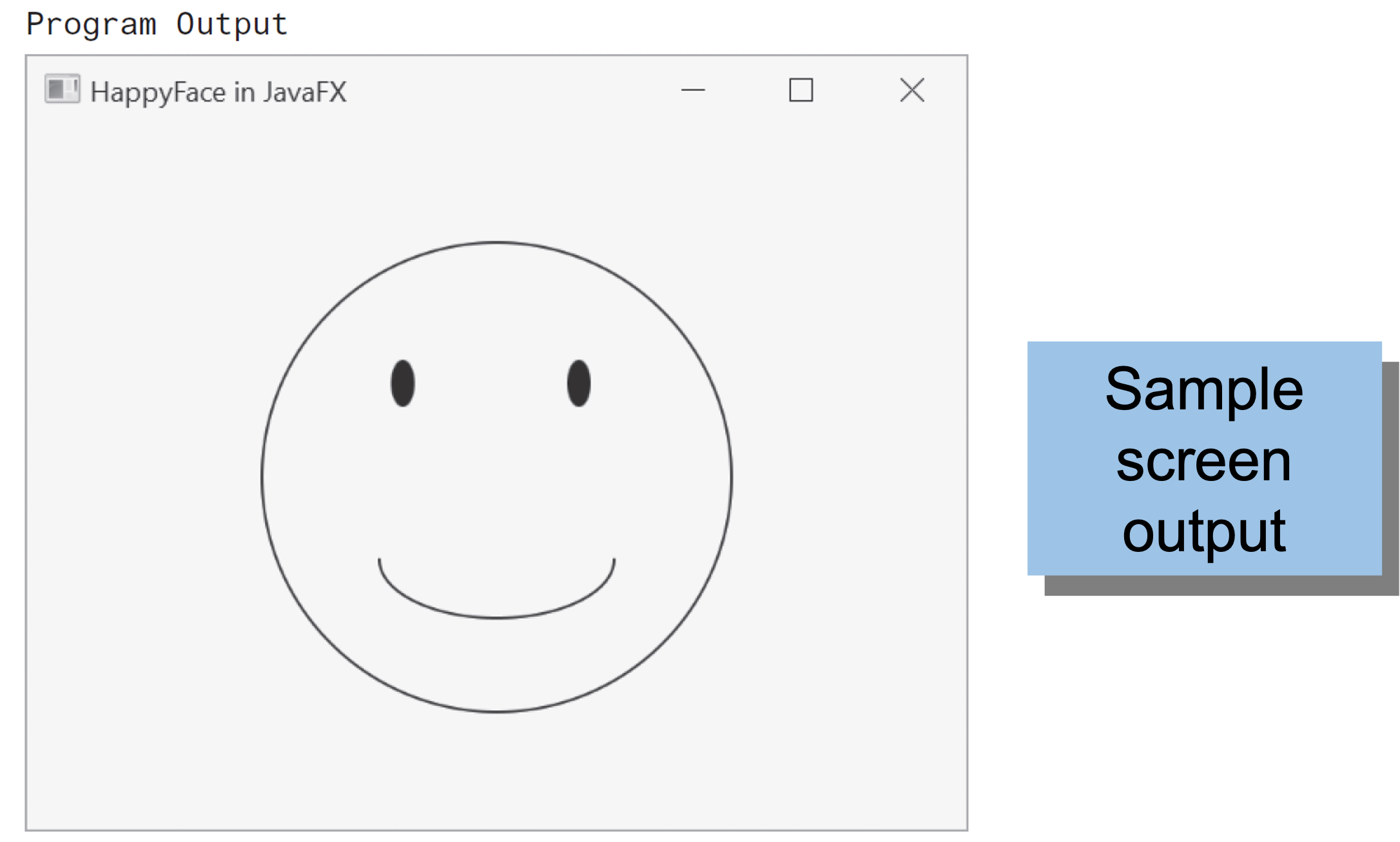
- Named constants makes it easier to find values.
- Comments and named constants male changing the code much easier.
- Named constants protect against changing the wrong.
JOptionPane
- View sample program class
JOptionPaneDemo
, listing 2.10
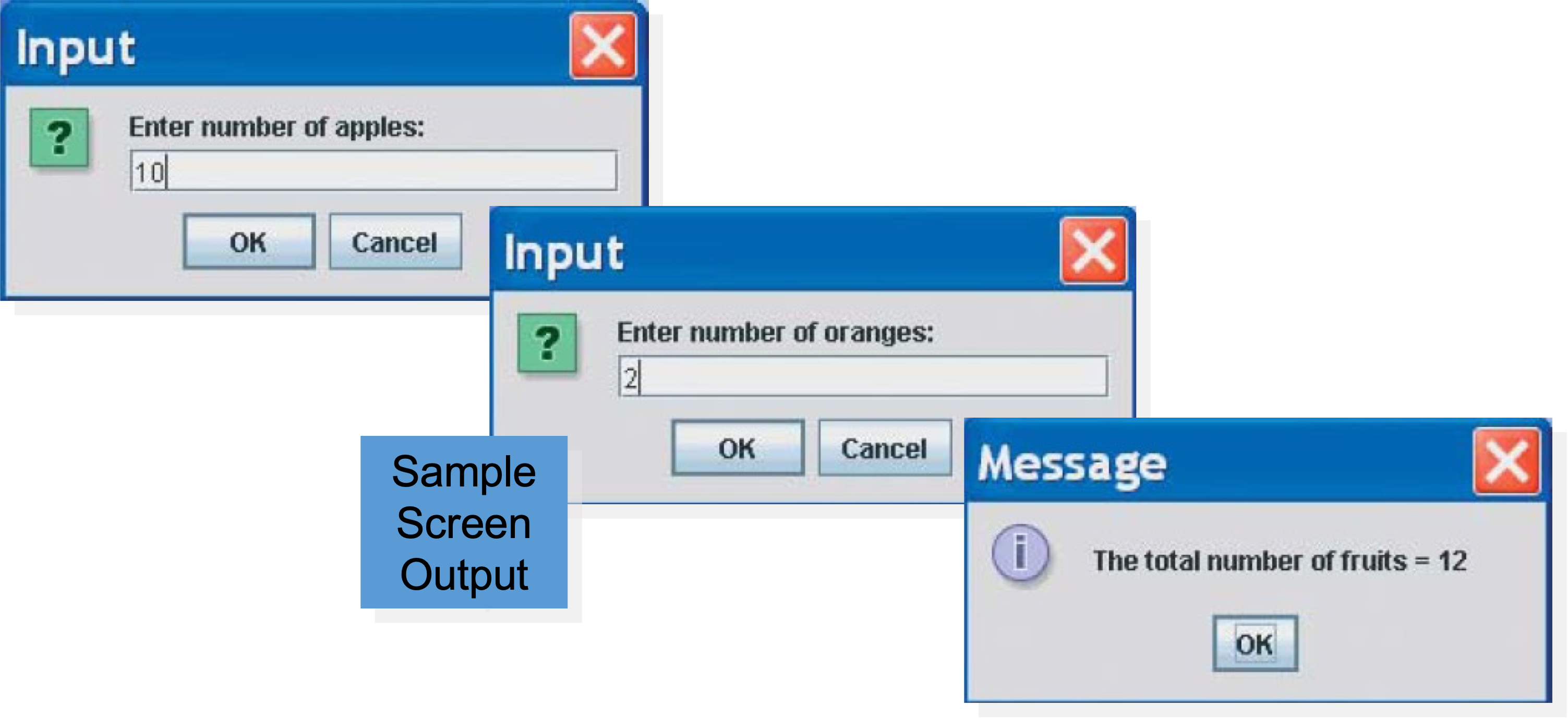
-
JOptionPane
can be used to construct windows that interact with the user.
- The
JOptionPane
class is imported by
import javax.swing.JApplet;
- The
JOptionPane
class produces windows for obtaining input or displaying output.
- Use
showInputDialog()
for inout.
- Only string values can be input.
- To convert an input value from a string to an integer use the
parseInt()
method from the Integer
class, use
appleCount = Integer.parseInt(appleString);
- Output is displayed using the
showMessageDialog
method.
JOptionPane.showMessageDialog(null, "Total number of fruits = " + totalFruitCount);
- Syntax
- Input
String Variable = JOptionPane.showInputDialogue (String_Expression);
- Output
JOptionPane.showMessageDialog (null, String_Expression);
-
Sytem.exit(0)
ends the program.
JOptionPane
Cautions
- If the input is not in the correct format, the program will crash.
- If you omit the last line (
System.exit(0)
), the program will not end, even when the ok
button in the output window is clicked.
- Always label any output.
-
JOptionPane.showInputDialog
can be used to input any of the numeric types.
- Figure 2.8 Methods for converting string to numbers
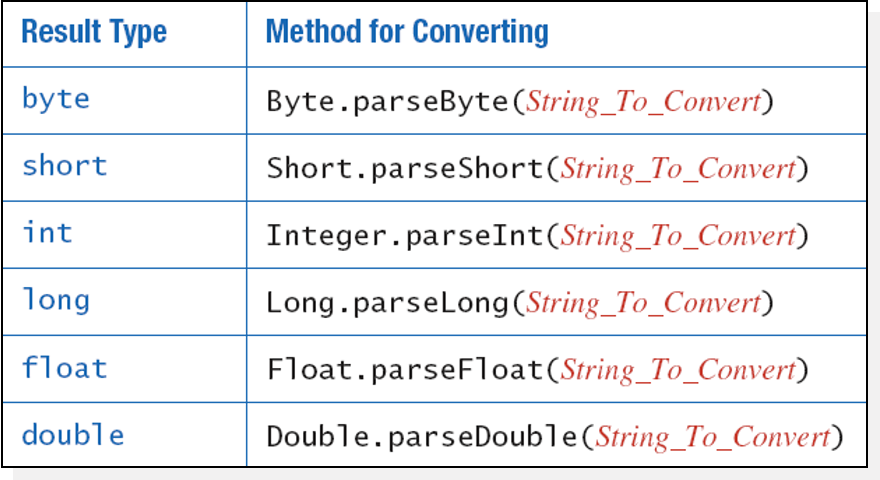
Mult-Line Output Windows
- To output multiple lines using the method
JOptionPane.showMessageDialog
, insert the new line character '\n'
into the string used as the second argument.
OptionPane.showMessageDialog(null, "The number of apples\n" + "plus the number of oranges\n" + "is equal to " + totalFruit);
- Figure 2.9 A dialog window containing multiline output
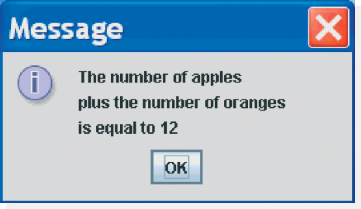
Programming Example
- View sample program class
ChangeMakerWindow
, listing 2.11
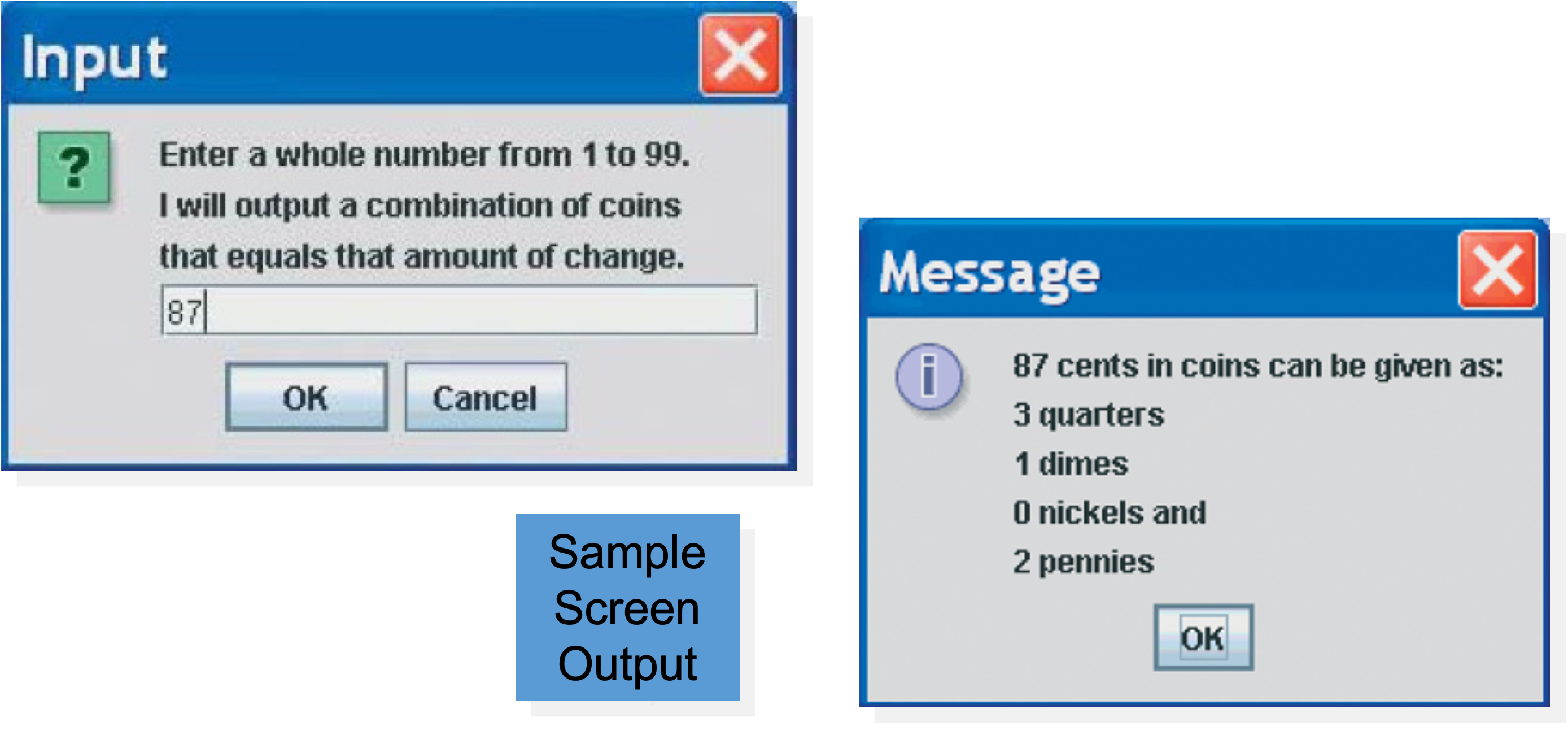
Graphics Supplement: Outline Section 3.4
Specifying a Drawing Color
- When drawing a shape inside a JavaFX canvas think of the drawing being done with a pen that can change colors.
- The method
setFill
changes the color of the “pen”
gc.setFill(Color.YELLOW);
- Drawings done later appear on top of drawings done earlier.
- View sample program, Listing 3.6 class
YellowFace
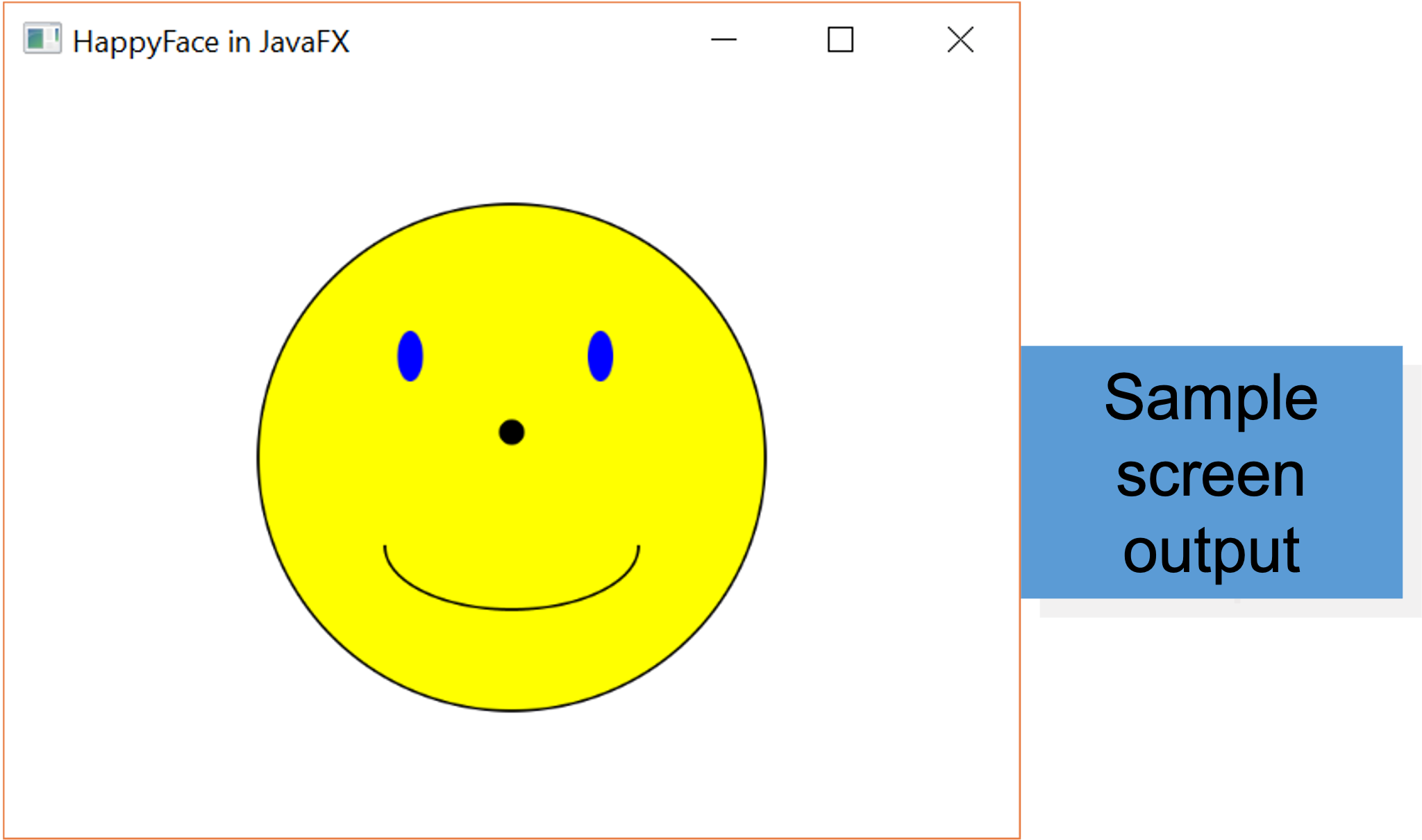
- Figure 3.10 Predefined Colors for the
setColor
method
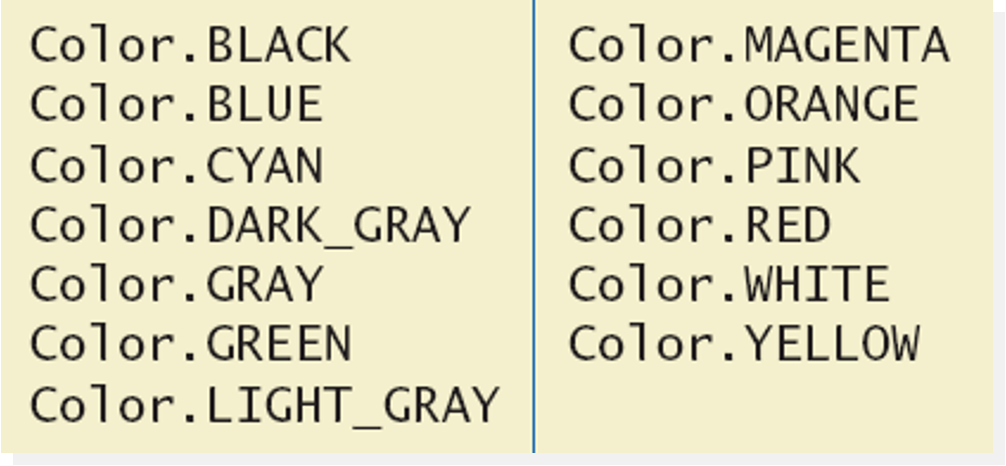
A Dialog Box for a Yes-or-No Question
- Used to present the user with a yes/no question
- The window contains
- The question text
- Two buttons labeled
yes
and no
.
- Example:
1
2
3
4
5
6
|
int answer = JOptionPane.showConfirmDialog(null, "End Program?", "Click Yes or No:", JOptionPane.YES_NO_OPTION);
if (answer == JOptionPane.YES_OPTION) {
System.exit(0);
} else if (answer == JOptionPane.NO_OPTION) {
System.out.println("One more time");
}
|
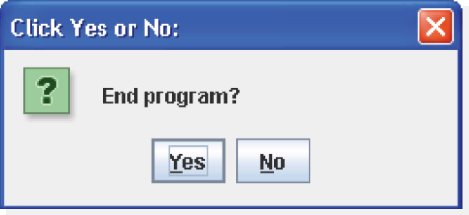
- Figure 3.11 A Yes-or No-Dialog Box
Programming Example
- A multiface JavaFX Application
- Uses loop to draw several smiley faces
- Uses if statement to alter appearance
- View sample program, listing 4.9 class
MultipleFaces
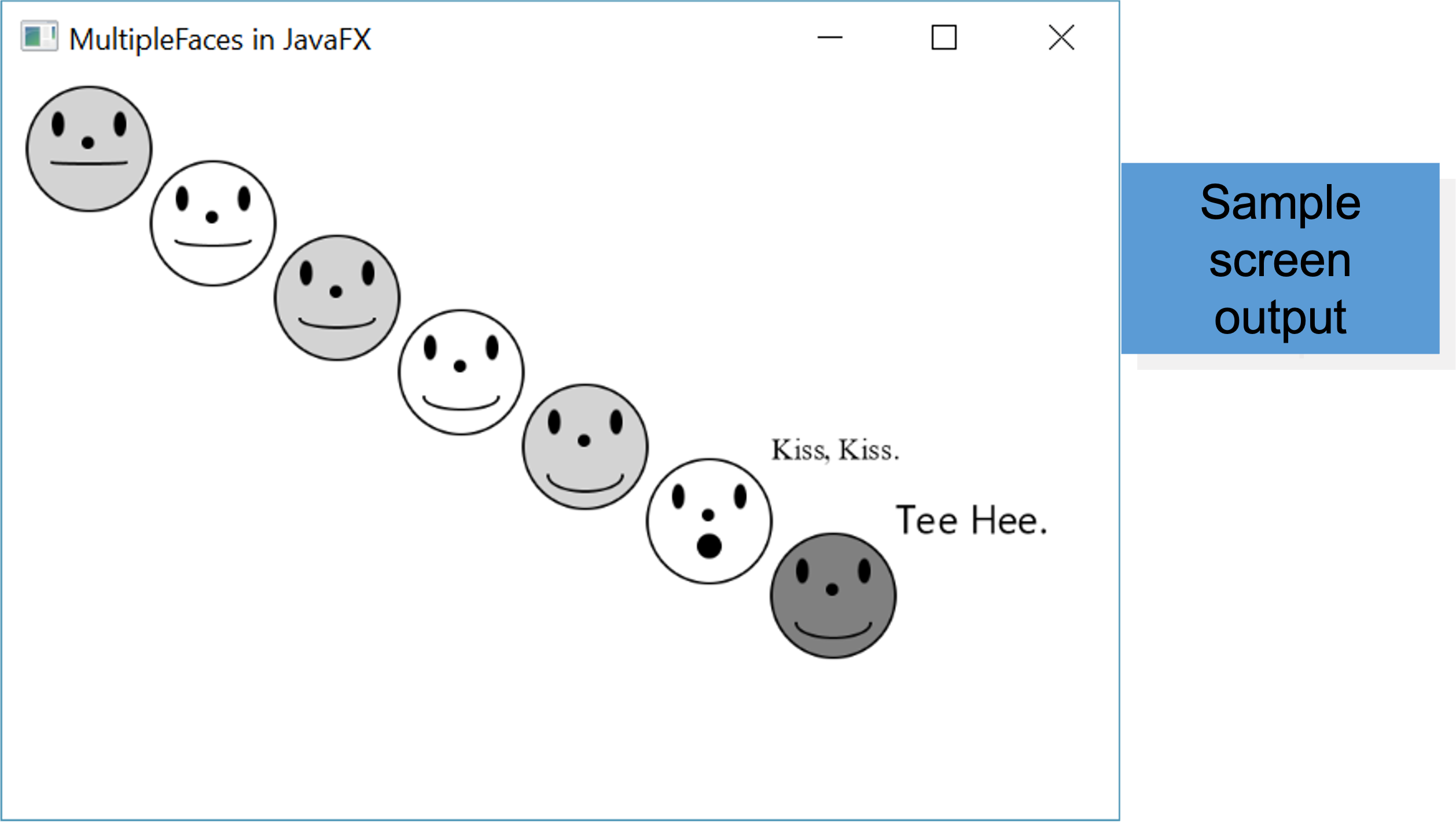
The fillText
Method
- Similar to methods for drawing ovals
- Displays Text
- Example
gc.fillText("Hello", 10, 20);
- Writes world hello at point (10, 20)
- Used to place “Kiss, Kiss” and “Tee Hee” on screen in listing 4.9
Powerpoint