Streams, File I/O, and Networking
Savitch 10.4-10.6
Overview
Objectives
- Describe the concept of an I/O stream
- Explain the difference between text and binary files
- Save data, including objects, in a file
- Read data, including objects, in a file
10.4 – Basic Binary-File I/O
Creating a Binary File
- Stream class
ObjectOutputStream
allows files which can store
- Values of primitive types
- Strings
- Other objects
- View program which writes integers, listing 10.5 class
BinaryOutputDemo
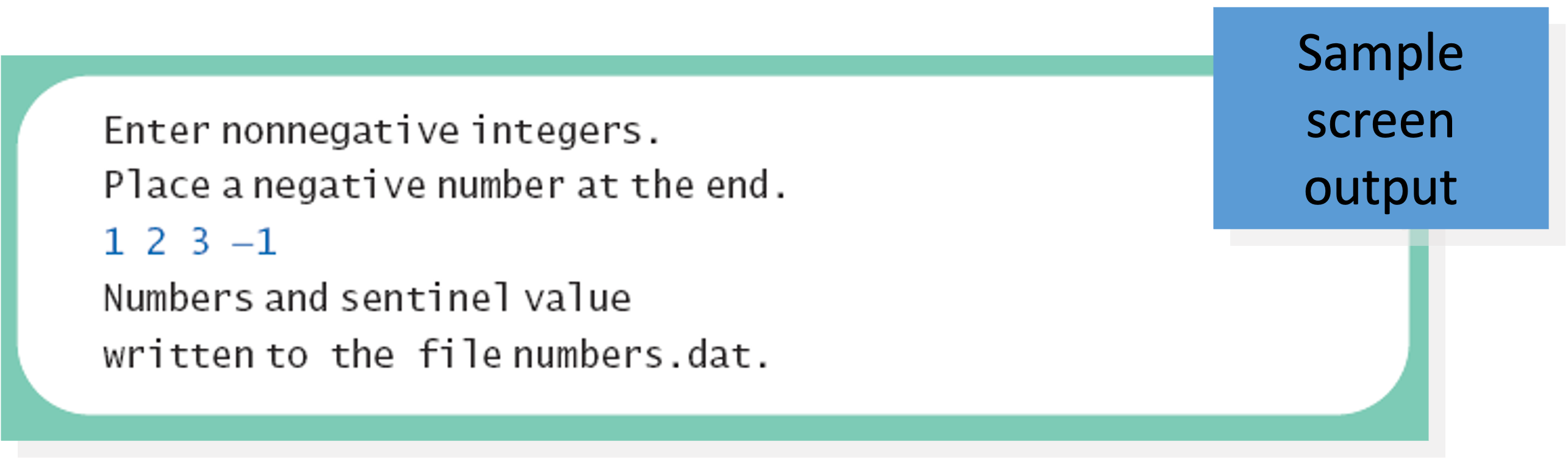
- Note the line to open the file
- Constructor for
ObjectOutputStream
cannot take a String
parameter
- Constructor for
FileOutputStream
can
Writing Primitive Values to a Binary File
- Method println not available
- Instead use writeInt Method
- View in listing 10.5
- Binary file stores numbers in binary form
- A sequence of bytes
- One immediately after another
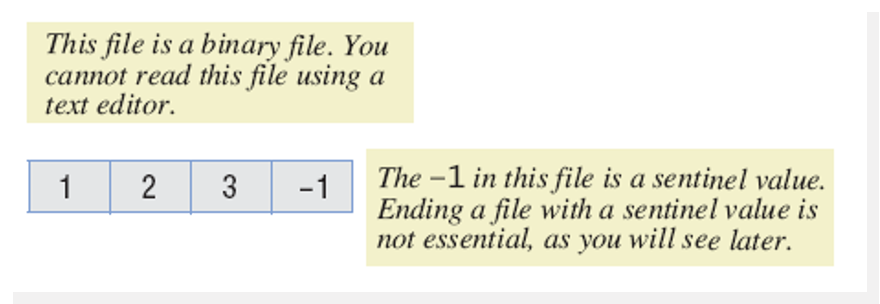
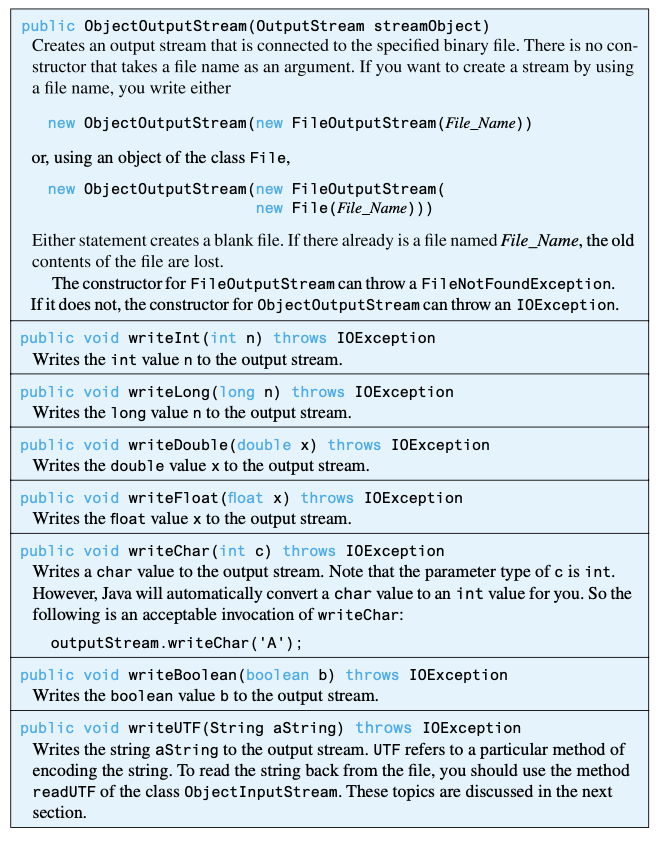
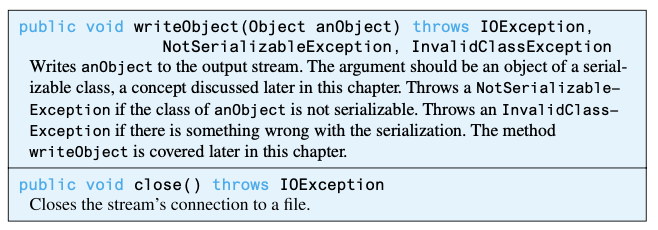
Writing Strings to a Binary File
- Use method
writeUTF
- Example:
1
|
outputStream.writeUTF("Hi Mom");
|
- UTF stands for Unicode Text Format
- Uses a varying number of bytes to store different strings
- Depends on length of string
- Constrast to
writeInt
which uses same for each
Reading from a Binary File
- File must be opened as an
ObjectInputStream
- Read from binary file using methods which correspond to write methods
- Integer written with
writeInt
will be read with readInt
- Be careful to read same type as was written
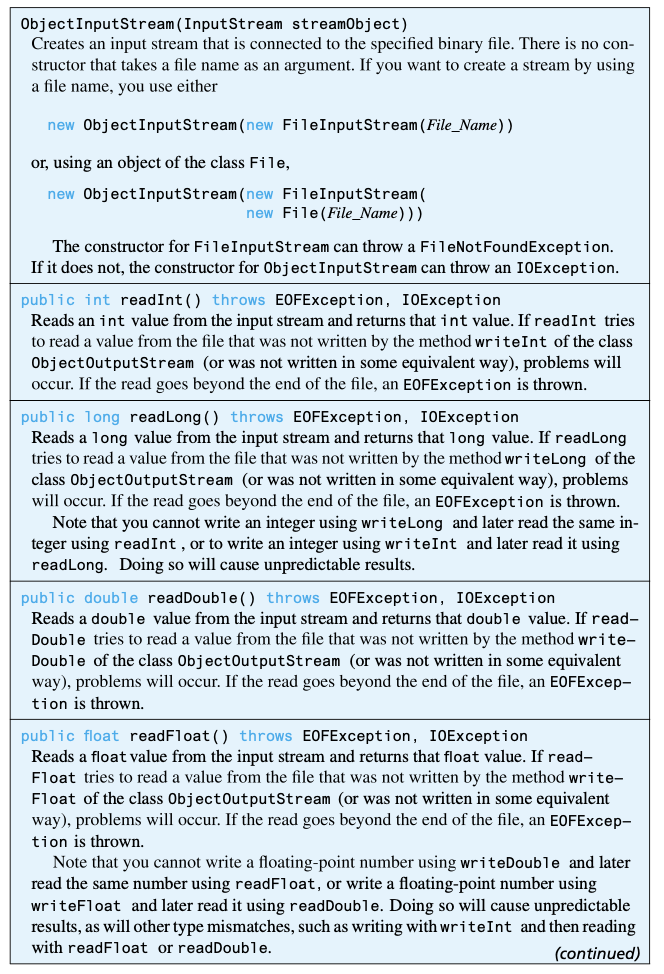
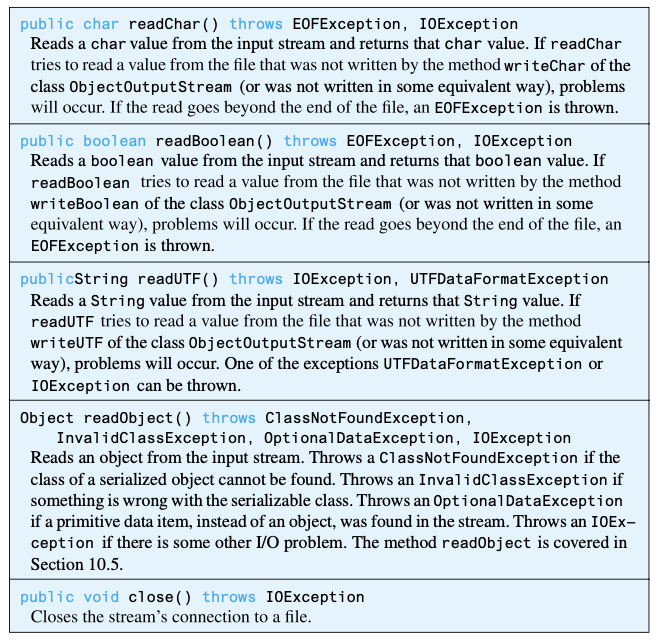
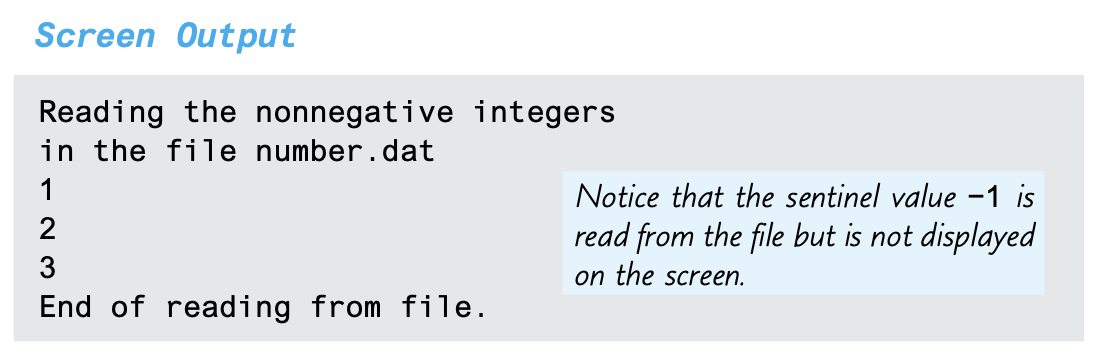
- View program to read, listing 10.6 class
BinaryInputDemo
The Class EOFException
- Many methods that read form a binary file will throw an
EOFException
- Can be used to test for end of file
- This can end a reading loop
- View example program, listing 10.7 class
EOFExceptionDemo
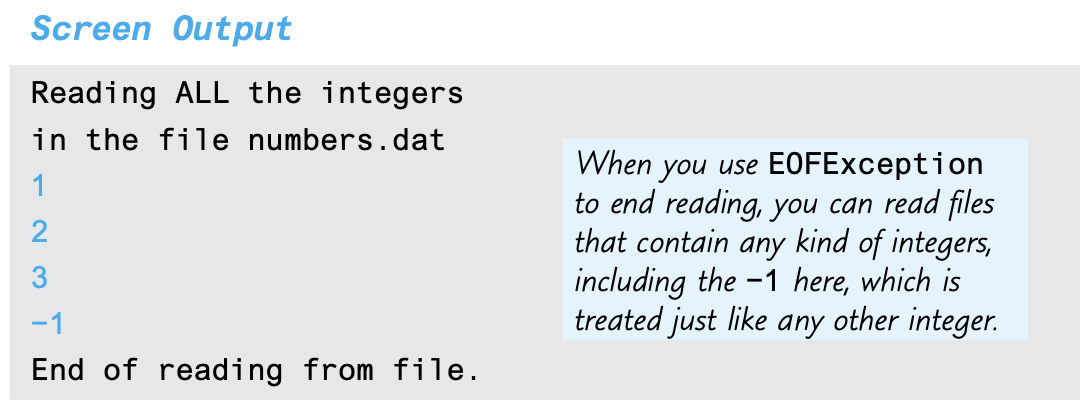
- Note the
-1
formerly needed as a sentinel value is now also read
- Always a good idea to check for end of file even if you have a sentinel value
Programming Example: Processing a File of Binary Data
- Processing a file of binary data
- Asks user for 2 file names
- Reads numbers in input file
- Doubles them
- Writes them to output file
- View processing program, listing 10.8 class
Doubler
10.5 – Binary-File I/O, Objects, & Arrays
Binary-File I/O with Class Objects
- Consider the need to write/read objects other than
Strings
- Possible to write the individual instances variables values
- Then reconstruct the object when file is read
- A better way is provided by Java
-
Object serialization - represent an object as a sequence of bytes to be written/read
- Possible for any class implementing
Serializable
- Interface
Serializable
is an empty interface
- No need to implement additional methods
- Tells Java to make the class serializable (class objects convertible to sequence of bytes)
- View sample class, listing 10.9 class
Species
- Once we have a class that is specified as
Serializable
we can write objects to a binary file
- Read objects with method
readObject();
- Also required to use typecast of the object
- View sample program, listing 10.10 class
ObjectIODemo
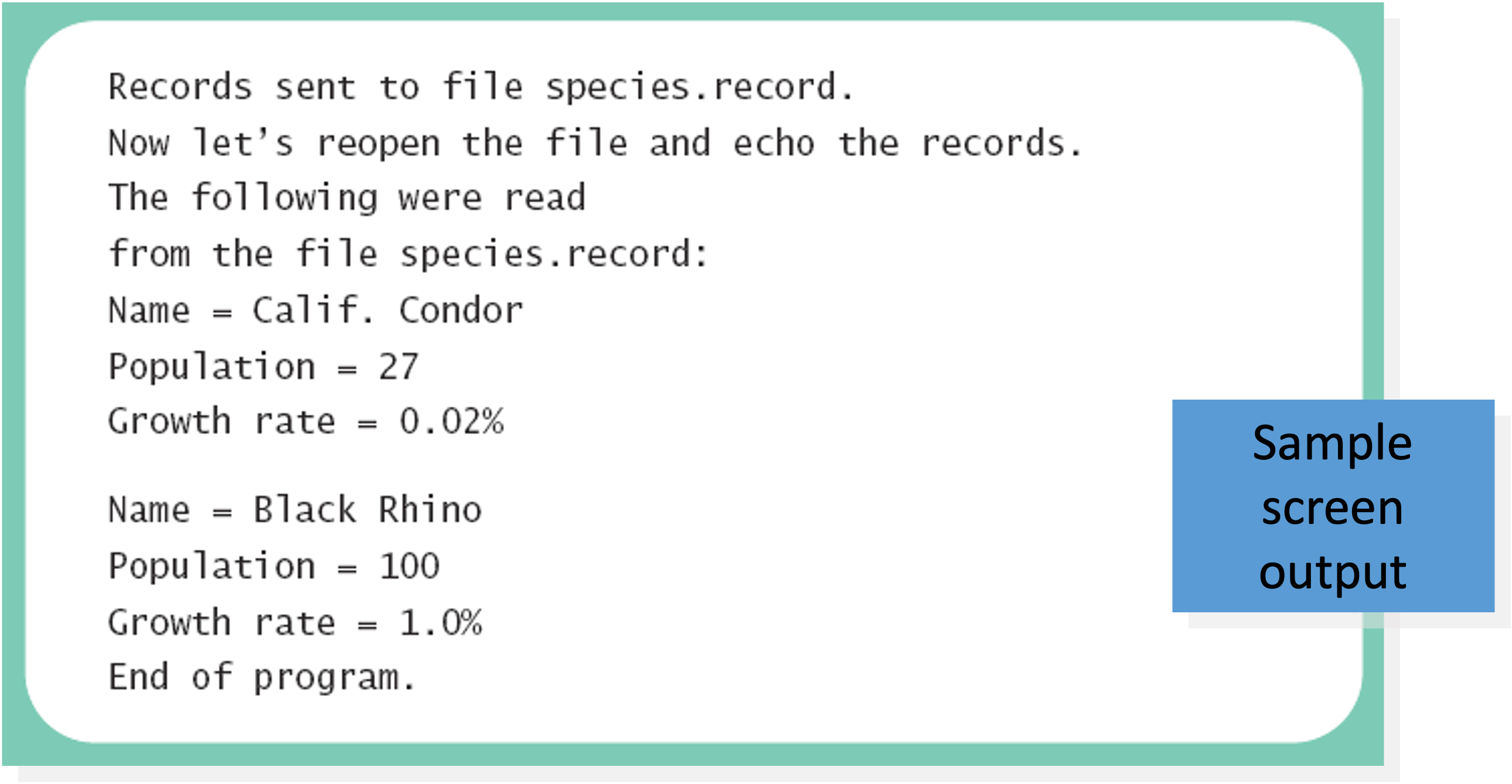
Some Details of Serialization
- Requirement for a class to be serializable
- Implements interface
Serializable
- Any instance variables of a class type are also objects of a serializable class
- Class’s direct superclass (if any) is either serializable or defines a default constructor.
- Effects of making a class serializable
- Affects how Java performs I/O with class objects
- Java assigns a serial number to each object of the class it writes to the
ObjectOutputStream
- If same object written to stream multiple times, only the serial number written after first time.
Array Objects in Binary Files
- Since an array is an object, possible to use
writeObject
with entire array
- Similarly use
readObject
to read entire array
- View array I/O program, listing 10.11 class
ArrayIODemo
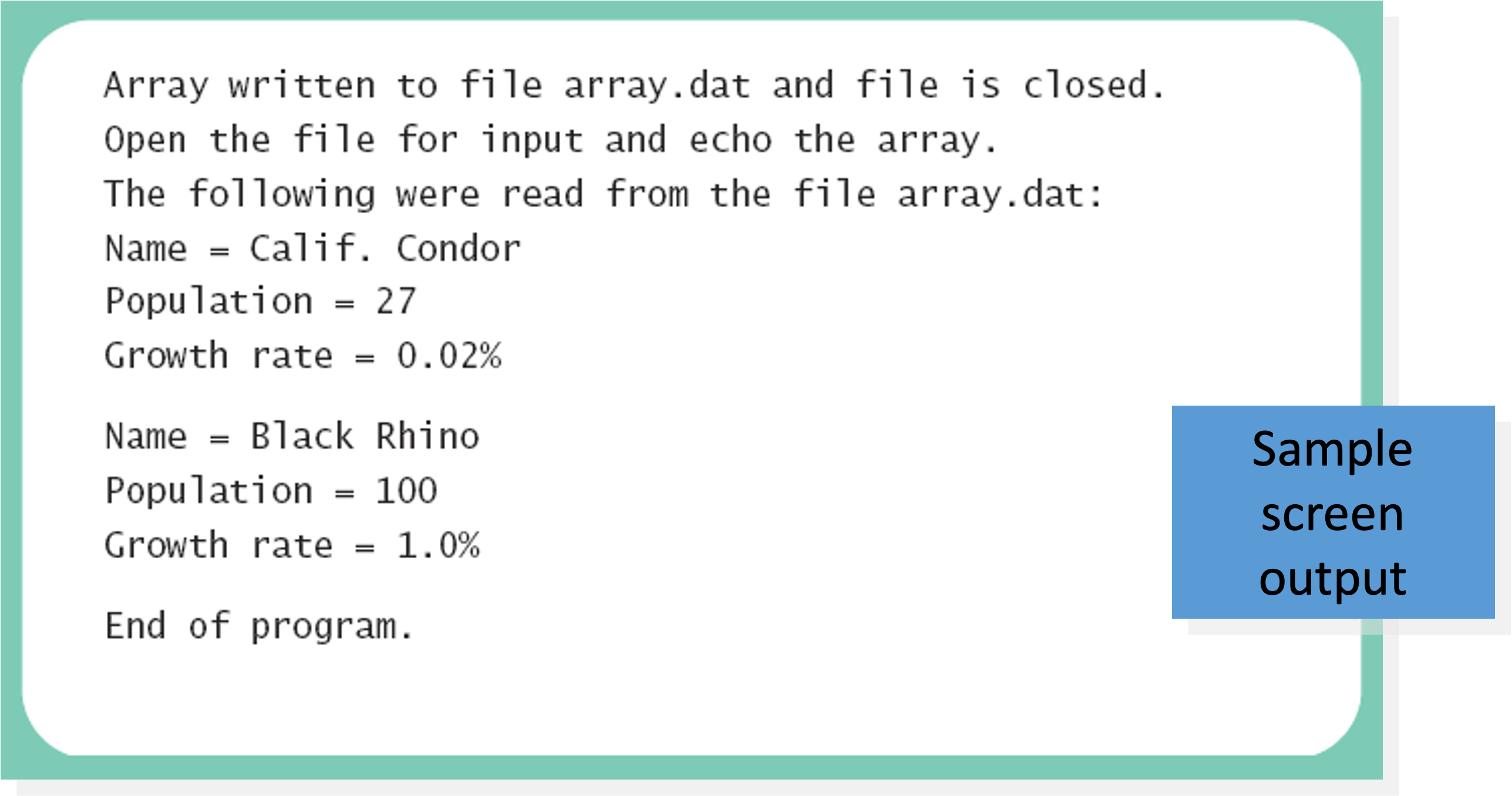
10.6 – Introduction to Sockets and Networking
- Classes such as Scanner and PrintWriter can be used with any data system - such as communicating over a network using streams.
- Java uses sockets
- A socket consist of the address that identifies the remote computer and a port ranging from 0 to 65535
- The process of communicating between a client and server is shown in the following figure
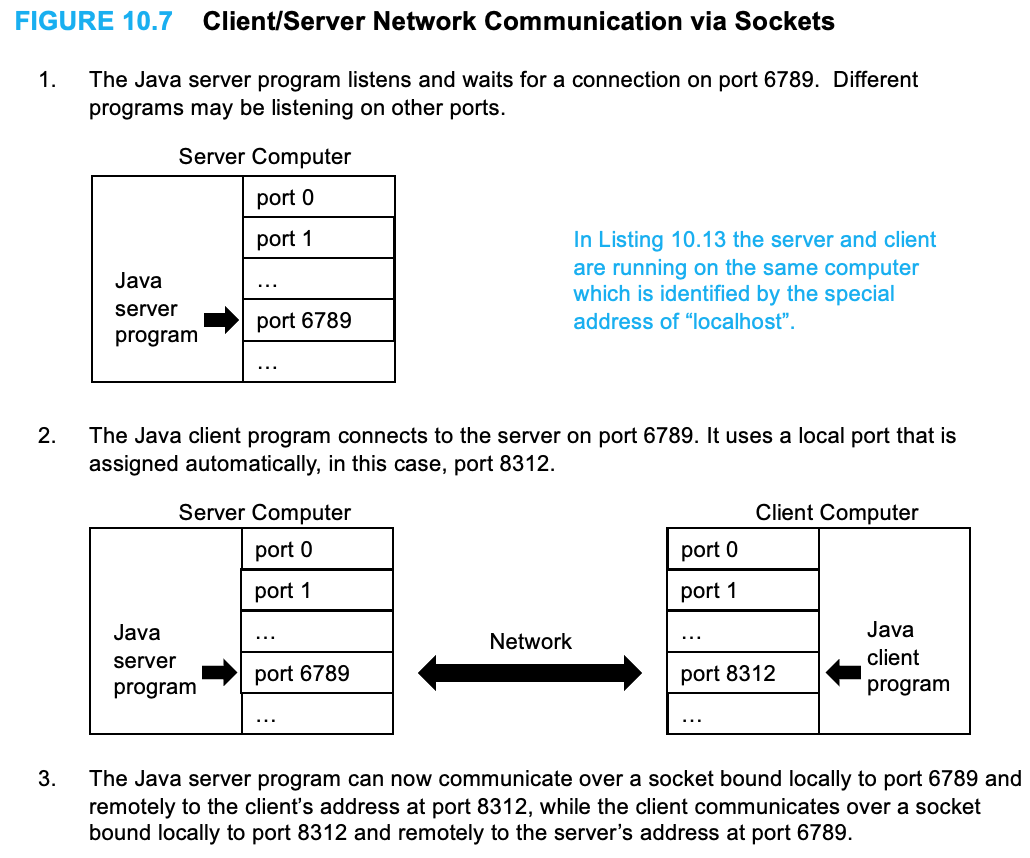
- Server Program
- Listen for a connection on a specified port; when one is made:
- Create a Scanner with an
InputStreamReader
based on the socket that the server will listen on; use this for input from a client
- Create a PrintWriter with the socket to send data to the client
- See listing 10.12
- Client Program
- Initiate a connection to the server on a specified port
- Create a Scanner to read from the socket
- Create a PrintWriter to send to the socket
- See Listing 10.13
The URL Class
- The URL class gives us a simple way to read from a webpage
- Thanks to polymorphism we can create a Scanner that is linked to a website
- The example outputs the text from wikipedia
1
2
3
4
5
6
7
8
9
|
URL website = new URL("https://www.wikipedia.org");
Scanner inputStream = new Scanner(new InputStreamReader(website.openStream)));
while (inputStream.hasNextLine())
{
String s = inputStream.nextLine();
System.out.println(s);
}
inputStream.close();
|
Powerpoint