Classes and Methods - Review
Savitch 5.1-5.3, 6.1-6.4
Topics
5.1 – Class and Method Definitions
Class and Method Definitions
- Objects in a program can represent real-world objects or abstract concepts.
- Every object is an instance of a class.
- A class specifies the attributes of an object and the actions an object can take.
- A UML class diagram can be used to summarize the properties of a class.
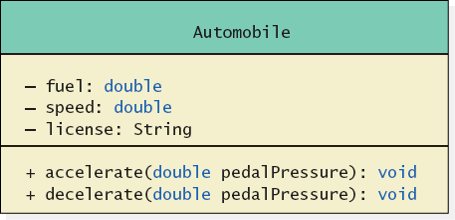
Instance Variables
Methods
Defining void
Methods
- A
void
method is a method that does not return a value.
- The method header contains the following information:
- The access modifier:
public
- The return type:
void
- The name of the method:
writeOutput
- The parameter list:()
- The method body contains a sequence of statements to execute when the method is called, and must be surrounded by
{
and }
.
Defining Methods That Return a Value
-
A method can be defined to return a value.
1
2
3
4
5
6
7
8
9
|
public int getAgeInHumanYears() // return type is int
{
int humanAge = 0; // local variable
if (age <= 2)
humanAge = age * 11;
else
humanAge = 22 + ((age – 2) * 5);
return humanAge; // return statement
}
|
-
When the method is called, the return value should be stored in a variable.
1
|
int humanYears = myDog.getAgeInHumanYears();
|
The Keyword this
Local Variables and Blocks
- Any variable declared inside of a method body is local to that method and can not be accessed outside of it.
- Two different local variables declared in different methods can have the same name.
- Any variable declared inside of a block (in between a
{
and a }
) is local to that block and can not be accessed outside of it.
- A variable inside of a block and a variable outside a block can have the same name.
Parameters of a Primitive Type
-
Parameters are variables that are defined in a method header and can be used in the method body.
1
2
3
4
5
6
|
public void initialize(String name, String breed, int age)
{
this.name = name;
this.bread = breed;
this.age = age;
}
|
-
For each parameter defined in a method header, an argument must be provided when the method is called.
1
|
myDog.initialize("Cheetah", "Australian Cattle Dog", 2);
|
-
Information hiding means that a programmer using a method needs to know what the method does, not how it does it.
- Comments can be used to specify what a method does.
- A precondition comment states the conditions that must be true before the method is called.
- A postcondition comment describes all of the effects produced by a method call.
The public
and private
Modifiers
- Java uses the access modifiers
public
and private
to specify how a class, method, or instance variable can be accessed.
- A public method or instance variable is accessible to a method of any other class.
- A private method or instance variable is only accessible to methods in the same class.
- Methods and named constants used outside of the class should be public.
- Instance variables and methods only used inside the class should be private.
Accessor Methods and Mutator Methods
Methods Calling Methods
- A method body can contain a call to another method.
- If the method being called is in the same class, the receiving object does not need to be specified.
1
2
3
4
5
6
7
8
9
10
|
public void displayMessage()
{
System.out.println("Hello from displayMessage!");
displayRestOfMessage(); // same as this.displayRestOfMessage()
}
private void displayRestOfMessage()
{
System.out.pritnln("Hello from displayRestOfMessage!");
}
|
Encapsulation
-
Encapsulation means that the details of a class are hidden if they are not necessary to understand how objects of the class are used.
- The class interface consists of the headers of the public methods and any public named constants in the class.
- The implementation of a class consists of the instance variables and method definitions in the class.
- A class is well encapsulated if changes to the implementation can be made without altering the class interface.
5.3 – Objects and References
Variables of a Class Type
Defining an equals
Method for a Class
Parameters of a Class Type
Chapter 5 Summary
6.1 – Constructors
Defining Constructors
- A constructor is a special method that is called whenever the
new
keyword is used.
- Constructors are typically used to initialize private instance variables.
- A constructor that takes no arguments is called a default constructor .
- If no constructor is defined, Java defines a default constructor that initializes the private instance variables to default values.
- Multiple constructors can be defined as long as they have a different number of parameters or have parameters with different types.
- Constructors with parameters must be provided arguments when invoked.
Example: Pet class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
public class Pet
{
private String name;
private int age;
private double weight;
// default constructor
public Pet()
{
name = "No name yet.";
age = 0;
weight = 0;
}
// constructor with parameters
public Pet(String name, int age, double weight)
{
this.name = name;
this.age = age;
this.weight = weight;
}
}
// Calls to constructors:
Pet pet = new Pet(); // calls default constructor
Pet myDog = new Pet("Cheetah", 2, 45); // calls 3-parameter
// constructor
|
Calling Methods from Constructors
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
public class Pet
{
private String name;
private int age;
private double weight;
public Pet()
{
set("No name yet.", 0, 0); // calls private method set
}
public Pet(String name, int age, double weight)
{
set(name, age, weight); // calls private method set
}
public Pet(String name)
{
set(name, 0, 0); // calls private method set
}
// initializes the private data members
private void set(String name, int age, double weight)
{
this.name = name;
this.age = age;
this.weight = weight;
}
}
|
6.2 – Static Variables and Static Methods
Static Variables
Static Methods
- A static method can be invoked without creating an object.
-
Static methods can be declared using the keywords static
.
1
|
public static void main (String args[]);
|
-
To access a public static method outside of a class, it must be preceded by the class name and the .
operator.
1
|
double root =Math.sqrt(2);
|
- Static methods can only access instance variables and non-static methods if another object of the class has been explicitly created.
The main
Method
The Math
Class
Wrapper Classes
- Java provides wrapper classes for each primitive type that contain methods specific to each type.
1
2
3
4
5
6
7
8
9
10
11
|
Integer num = new Integer(42); // Integer class constructor
Integer num = 42; // same as above, uses boxing to convert to object
int ival = num.intValue(); // converts back to an int
int ival = num; // same as above, uses unboxing to convert to int
double num = Double.parseDouble("3.14159"); // converts to double
char ch = Character.toUpperCase('a'); // converts to upper case
|
6.3 – Writing Methods
Decomposition
-
Decomposition is the process of breaking a task into subtasks.
- Example: display double
amount
as dollars and cents
- Set
dollars
to the number of whole dollars in amount.
- Set cents to the number of cents in
amount
, rounded to two decimal places.
- Display a dollar sign,
dollars
, and a decimal point.
- Display cents as a two digit integer.
- Each task can be solved separately, then converted to code.
- Solutions to subtasks can be implemented as separate private methods.
Addressing Compiler Concerns
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
public static int badMax(int val1, int val2)
{
if (val1 > val2) // This code will not compile because
return val1; // it does not cover the case where
else if (val2 > val1) // val1 == val2
return val2;
}
public static int goodMax(int val1, int val2)
{
int answer = val1; // Creating a variable for the return value
if(val2 > val1) // and using a single return statement
answer = val2 // guarantees that a value will be returned
return answer;
}
|
The null
Constant
-
If a variable of a class type needs to be initialized, the constant null
can be used.
- The
null
constant is a placeholder that does not refer to an actual object.
-
Variables of class type may be compared with null using ==
and !=
.
1
2
|
if (line == null)
...
|
- If the
.
operator is used on a variable with a value of null
, it will generate a null pointer exception and halt the program.
Testing Methods
6.4 – Overloading
Overloading Basics
- A method name is overloaded if there are two or more methods in the same class with that name.
- The signature of a method consists of its name and parameter types.
- Methods in the same class with the same name must have different signatures.
1
2
3
4
5
6
7
8
9
|
public double getAverage(double first, double second)
{
return (first + second) / 2.0;
}
public double getAverage(double first, double second, double third)
{
return (first + second + third) / 3.0;
}
|
Overloading and Automatic Type Conversion
- Automatic type conversion can interfere with overloading.
-
Consider the following overloaded methods:
1
2
|
public static void problemMethod(double n1, int n2) { ... }
public static void problemMethod(int n1, double n2) { ... }
|
-
These methods are legal, but the following call will generate a compiler error:
1
|
problemMethod(5, 10); // could invoke either method
|
- An
int
can be automatically converted to a double
.
- The compiler can not determine which method to use.
Overloading and the Return Type
Chapter 6 Summary
- A constructor is a method that creates and initializes an object of the class when you invoke it using the operator
new
. A constructor must have the same name as the class.
- A constructor without parameters is called a default constructor. A class that does not define a constructor will be given a default constructor automatically. A class that defines one or more constructors, none of which is a default constructor, will have no default constructor.
- When defining a constructor for a class, you can use
this
as a name for another constructor in the same class. Any call to this
must be the first action taken by the constructor.
- The declaration of a static variable contains the keyword
static
. A static variable is shared by all the objects of its class.
- The heading of a static method contains the keyword
static
. A static method is typically invoked using the class name instead of an object name. A static method cannot reference an instance variable of the class, nor can it invoke a non-static method of the class without using an object of the class.
- Each primitive type has a wrapper class that serves as a class version of that primitive type. Wrapper classes also contain a number of useful predefined constants and methods.
- Java performs an automatic type cast from a value of a primitive type to an object of its corresponding wrapper class—and vice versa—whenever it is needed.
- When writing a method definition, divide the task to be accomplished into subtasks.
- Every method should be tested in a program in which it is the only untested method.
- Two or more methods within the same class can have the same name if they have different numbers of parameters or some parameters of differing types. That is, the methods must have different signatures. This is called overloading the method name.
- An enumeration is actually a class. Thus, you can define instance variables, constructors, and methods within an enumeration.
- You can form a package of class definitions you use frequently. Each such class must be in its own file within the same folder (directory) and contain a
package
statement at its beginning.
Powerpoint
Quiz
Quiz 1